Azure functions cron job
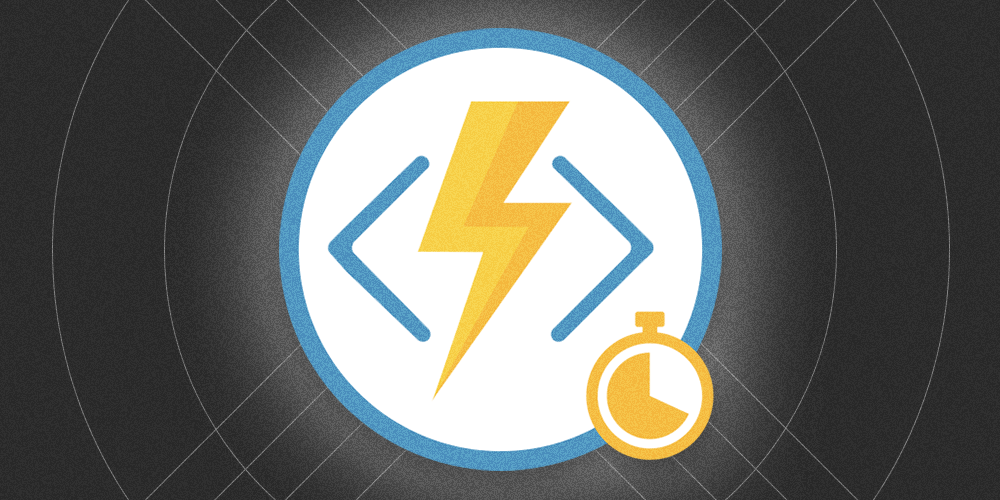
Azure function cron job
Azure functions can be used in countless different ways. One of these is setting up a Cron timer as the trigger for your function, allowing it to run in a scheduled manner. In this post I will show you how to create a cron job with Azure functions.
Let's get started by opening up Visual Studio 2022 and selecting the Azure Functions template. I'm using .NET 6.0 (LTS) here, but any recent version of .NET will do. As function trigger we will select a Timer trigger.
Image: Creating a new functions project in Visual Studio 2022
Don't worry about getting the initial settings wrong, as they can all be changed later. When creating the project we get the following starting point:
The Run
method is the entry point for our function. The TimerTrigger
attribute is what triggers our function. The string value is a cron expression that determines when the function will be executed. In this case the function will run every 5 minutes. The TimerInfo
parameter contains information about the timer that triggered the function. The ILogger
parameter is used to log information to the Azure function log. Both the TimerInfo and ILogger are provided by means of dependency injection.
To evaluate the CRON expression, Azure Functions uses NCron under the hood. The expression consists out of 6 parts, separated by a space. The first 5 parts are the time parts, and the last part is the day of the week. The time parts, from left to right, are as follows:
Part | Values |
---|---|
Second | 0-59 |
Minute | 0-59 |
Hour | 0-23 |
Day of the month | 1-31 |
Month | 1-12 |
Day of the week | 0-6 |
The first part (the seconds) is optional, and can be omitted. Besides numbers or strings, we can also use the following characters:
Character | Description | Example |
---|---|---|
* | any value | Value doesn't matter |
, | value list separator | Combine multiple options |
- | range of values | Specify a range of sequential values |
/ | step values | Every nth value |
Multiple online resources exist to help you create a cron expression.crontab.cronhub.io for example, allows you to generate, test and verify cron expressions.
A practical example
Let's say I want to rebuild my blogging website from source, every morning at 6:00 AM. We can use the following expression to achieve this:
We modify our function to be Async, and create a new method that does the required work. In my case, this blog is hosted on Vercel , which allows me to call an HTTP endpoint to manually start the build process.
Deployment hooks can be created via the Vercel dashboard under Project > Settings > Git > Deploy Hooks
.
The actual value of the hook is stored as an environment variable in this case.
This way, our function will be triggered every morning at 6:00 AM, and will trigger a new build on Vercel!
Note
For this particular use case, I might make more sense to use the recently released Vercel cron jobs .
I wrote a blogpost about achieving the same result using Vercel cron jobs.