Svelte transitions
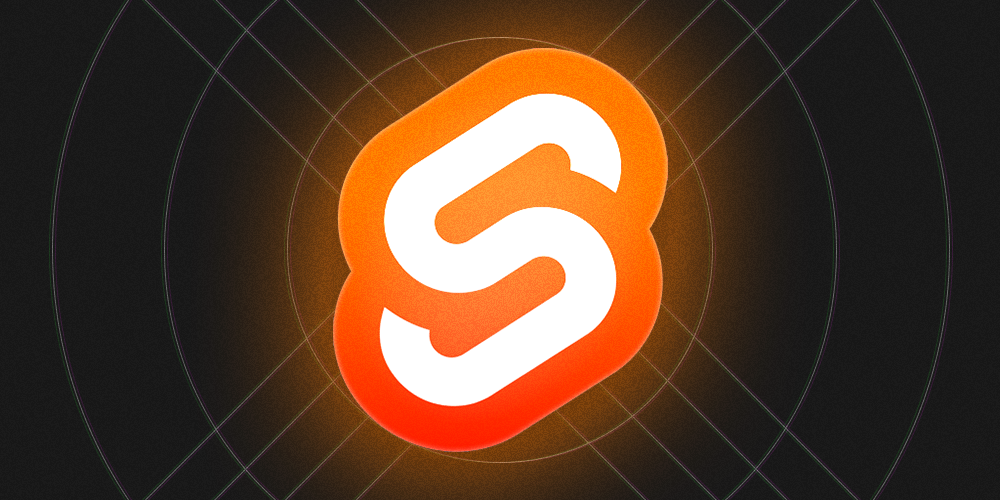
Svelte transitions
Svelte allows us to easily animate elements in and out of the DOM. A transition is an animation that plays when the element get created or deleted. They work in both directions, meaning that the animation that plays on deletion will be the inverse of the animation that plays on creation. To to achieve all of this this, we can make use of the transition directive.
Basic example
We can add the transition directive to native dom elements, such as a paragraph tag in this example:
Image: The paragraph fades in an out when the DOM elements get added or removed
Out-of-the-box transitions
At the time of writing, Svelte comes with a couple of transitions out-of-the-box:
- blur
- fade
- fly
- slide
- scale
- draw
- crossfade
Image: Fade
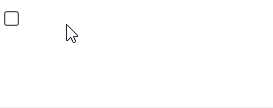
Image: Blur
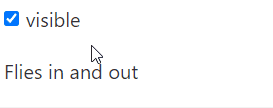
Image: Fly
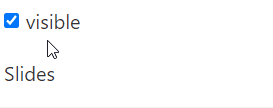
Image: Slide
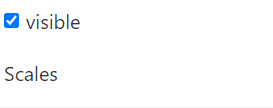
Image: Scale
The draw transition is a special one, as it can only be applied to an SVG path.
The following inline SVG drawing of a cross gets drawn by the transition
Image: Draw
Some of these transitions require additional parameters to work. All available options can be viewed under the Transitions source code on Github. As an example, the Fly transition can be given the y parameter (in pixels) and the duration (in ms).
Custom transitions
While these transitions probably cover most use cases, we might someday require a custom transition. We can define these custom transition function as follows:
The function takes in two arguments:
- node
- options object
The first one is the node that is the subject of the transition. The second argument is an object that contains any options you want to pass to the transition function, such as the duration, direction, color, ...
The function needs to return an object that can contain the following properties:
- delay: The duration (in ms) before the transition begins
- duration: The total duration of the transition
- easing: An (input : number) => output : number function that maps the input between [0,1] to the output [0,1], but with a tweening function.
- css: A function (t,u) = {..} that returns a string containing the relevant css for the node. Parameter t goes from 0,1, while parameter u goes from 1 to 0. t = u - 1 for the entire duration of the function
- tick: Similar to the css function in the shape of (t,u) => {..} that can modify the node. Usually not needed, as the css function should be enough to modify the styling of the node.
A typical css function looks as follows:
The function above would generate a CSS animation that changes the font size from 0rem to 1rem when the element gets created, and from 1rem to 0rem when destroying the object. This example only changes the font-size, but as many css properties as needed can be modified during a transition.
Image: Custom font-size transition
Layout transitions
Up until this point we have been applying transitions to single elements on a page. Of course we can also apply them to divs or other container elements.
But it gets even more interesting when applying transitions in a layout file. When we create a +layout.svelte file, we can apply a transition in the following way:
This will fade the entire content slot in and out. We add the absolute position tag to the div so that both slots are rendered on top of each other. This will prevent popping in when the original div disappears from the DOM.
When using the code as shown above, we get the following result:
Image: Layout fly transition
This works great! All the content in the slot tag flies in and out when a new page gets loaded.Directional transition
Now in case of the fly transition, we might want to detect the 'direction' of the transition. By this I mean that it could be useful to detect if we are moving from a deeply nested page to a more shallow nested page. For example:
- moving from 'demo/010' to 'demo/010/1' would be considered moving deeper, or moving right
- moving from 'demo/010/1' to 'demo/010' would be considered moving more shallow, or moving left
In the +layout.svelte file, we can determine the transition direction to be either LEFT or RIGHT be looking at the path depth. The depth is determined in the layout by using the 'beforeNavigate' hook.
We can use this direction in the same layout file to generate the options object that gets passed on to the fly transition, like so:
And by doing so, we reverse the transition direction going back up the url tree.
Image: Layout fly transition
Getting creative
The Batman transition
All of this of course leads to one single goal: the batman transition. The original batman series featured a spinning bat logo and matching sound effect. I've recreated this as a Svelte page transition. The sound is played by the afterNavigate hook, and a custom transition scales and rotates the logo when the url changes.
Image: I have peaked as a web developer
Accessibility
As mentioned in this great blogpost by Geoff Rich, we need to make sure that we keep the accessibility of our webpage in mind. Its easy to go wild with all these easy-to-use animations, but at the end of the day we want our website to be usable to all users.
The following css media query at the root level in our app.html file will detect if the user prefers reduced motion. If this is the case, we will disable all animations across the entire website.
As a reminder, you can enable this setting on your device or browser in the following way:
- In GTK/Gnome, if gtk-enable-animations is set to false. This is configurable via GNOME Tweaks (Appearance tab or General tab, depending on version). Alternately, add gtk-enable-animations = false to the Settings block of the GTK 3 configuration file (~/.config/gtk-3.0/settings.ini).
- In Windows 10: Settings > Ease of Access > Display > Show animations in Windows.
- In Window 7 [& 8]: Control Panel > Ease of Access > Make the computer easier to see > Turn off all unnecessary animations (when possible).
- In macOS: System Preferences > Accessibility > Display > Reduce motion.
- In iOS: Settings > General > Accessibility > Reduce Motion.
- In Android 9+: Settings > Accessibility > Remove animations.
Information found on this StackOverflow question
Further reading
- Svelte.dev interactive transition tutorial
- Svelte included transitions source on Github
- Accessible Svelte transitions by Geoff Rich
- Batman on Wikipedia